CharInsert is an extension that enables you to provide editors with links they can click to insert snippets into wikitext. Some use cases for this include:
- Giving easy access to single special characters
- Providing preloaded copyable text for templates that may need to be used many times on a page
- Inserting an empty standard skeleton when a user starts a new page
Here’s an example of a page with several options to insert characters:
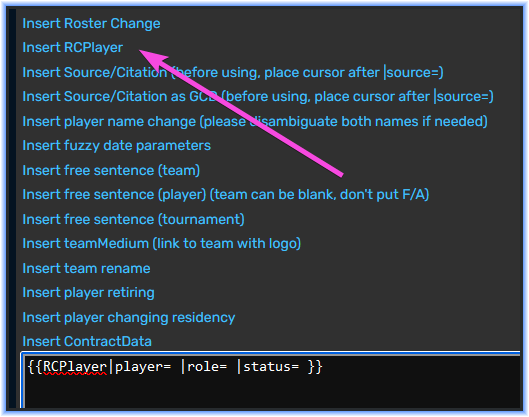
(Note: All code in this post was originally written by me for Leaguepedia and is licensed under CC BY-SA 3.0.)
Common inserts you might want to use
In the template namespace, you might want to add:
<charinsert><includeonly>+</includeonly><noinclude>{{documentation}}</noinclude></charinsert>
This will give you an insert that looks like this when you click it (notice the position of the cursor is where the +
is located in its definition):
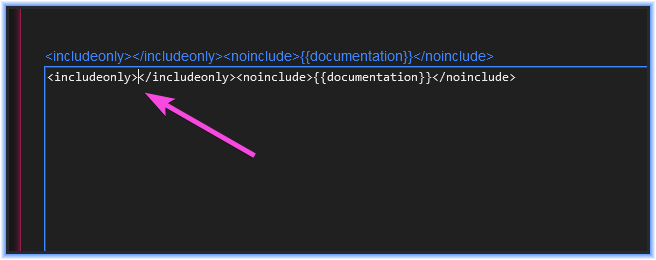
By default, spaces result in separate individual inserts, so to get a bunch of special characters you could do this:
<charinsert>Á á Ć ć É é Í í Ĺ ĺ Ń ń Ó ó Ŕ ŕ Ś ś Ú ú Ý ý Ź ź</charinsert>
In this case, we can click the “Á” to print Á
, etc. If you want literal space characters in your preload, you can also escape the whitespace with <nowiki> </nowiki>
tags.
A quick setup guide
The extension
CharInsert is available for install and documented on mediawiki.org.
Out of the box, the extension leaves a bit to be desired, so we’ll customize it with some on-wiki code.
CSS
Copy this to a CSS page on your wiki (MediaWiki:Common.css or a gadget, etc):
1
2
3
|
.client-js .mw-charinsert-item {
display:none;
}
|
JS
Copy this to a JS page on your wiki (MediaWiki:Common.js or a gadget, etc):
1
2
3
4
5
6
7
|
$(function() {
$('.mw-charinsert-item').each(function() {
$(this).html($(this).closest('div').attr('data-ci-label'));
$(this).css('display', 'inline-block');
});
$('.ci-loading-text').css('display','none');
});
|
Lua
There are two modules to import, Module:CharInserts
and one dependency:
Module:CharInserts
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
|
local util_table = require('Module:TableUtil')
local h = {}
local p = {}
function p.main(frame)
local title = mw.title.getCurrentTitle()
return h.makeOutput(h.getData(title.nsText), title.text)
end
function h.getData(nsText)
local moduleTitle = mw.title.new(h.getModuleName(nsText), 'Module')
if not moduleTitle.exists then return nil end
return mw.loadData(moduleTitle.prefixedText)
end
function h.getModuleName(nsText)
if nsText == '' then nsText = 'Main' end
return ('CharInserts/%s'):format(nsText)
end
function h.makeOutput(data, title)
if not data then return end
local ret = {}
for _, group in ipairs(data) do
ret[#ret+1] = h.getGroupOutput(group, title)
end
if #ret == 0 then
ret[#ret+1] = '<!-- -->'
end
return unpack(ret)
end
function h.getGroupOutput(group, title)
-- group.pattern is required to be in title
if not h.containsOneOf(title, group.pattern) then return nil end
-- group.notpattern is required NOT to be in title, and supercedes group.pattern being there
if h.containsOneOf(title, group.notpattern or '@@@@@@@@@@') then return nil end
-- newtext=true can be set to force the insert to show only in new pages
if group.newtext and mw.title.getCurrentTitle().exists then return nil end
local div = h.makeDiv(group.label)
h.printContent(div, group)
return div
end
function h.containsOneOf(title, patterns)
patterns = util_table.guaranteeTable(patterns)
for _, v in ipairs(patterns) do
if title:find(v) then
return true
end
end
end
function h.makeDiv(label)
return mw.html.create('div'):attr('data-ci-label', label)
end
function h.printContent(div, group)
div:wikitext(h.loadingText(group.label), h.makeContent(group.insert))
end
function h.loadingText(label)
local output = mw.html.create('span')
:addClass('ci-loading-text')
:wikitext('loading...')
return tostring(output)
end
function h.makeContent(insert)
return mw.getCurrentFrame():extensionTag{
name = 'charinsert',
content = h.makeReplacements(h.escape(insert)),
args = {}
}
end
function h.makeReplacements(str)
local replacements = {
PAGENAME = h.escape(mw.title.getCurrentTitle().text),
}
return h._doMakeReplacements(str, replacements)
end
function h._doMakeReplacements(sentence, replacements)
if not sentence then return nil end
if not replacements then replacements = {} end
local old = sentence
for k, v in pairs(replacements) do
sentence = sentence:gsub('%$' .. k .. '%$', v or 'unknown')
end
if sentence == old then
return sentence
end
return h._doMakeReplacements(sentence, replacements)
end
function h.escape(insert)
local replace = {
['\n'] = ' ',
['( +)'] = '<nowiki>%1</nowiki>',
['\\%['] = '[',
['\\%]'] = ']',
}
for k, v in pairs(replace) do
insert = insert:gsub(k, v)
end
return insert
end
return p
|
Module:TableUtil
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
311
312
313
314
315
316
317
318
319
320
321
322
323
324
325
326
327
328
329
330
331
332
333
334
335
336
337
338
339
340
341
342
343
344
345
346
347
348
349
350
351
352
353
354
355
356
357
358
359
360
361
362
363
364
365
366
367
368
369
370
371
372
373
374
375
376
377
378
379
380
381
382
383
384
385
386
387
388
389
390
391
392
393
394
395
396
397
398
399
400
401
402
403
404
405
406
407
408
409
410
411
412
413
414
415
416
417
418
419
420
421
422
423
424
425
426
427
428
429
430
431
432
433
434
435
436
437
438
439
440
441
442
443
444
445
446
447
448
449
450
451
452
453
454
455
456
457
458
459
460
461
462
463
464
465
466
467
468
469
470
471
472
473
474
475
476
477
478
479
480
481
482
483
484
|
local lang = mw.getLanguage('en')
local p = {}
function p.generalLength(tbl)
local n = 0
for _, _ in pairs(tbl) do
n = n + 1
end
return n
end
function p.getKeys(tbl, f)
local ret = {}
for k, _ in pairs(tbl) do
ret[#ret+1] = k
end
if f then table.sort(ret, f) end
return ret
end
function p.keyOf(tbl, val)
for k, v in pairs(tbl) do
if v == val then
return k
end
end
return nil
end
function p.hash(tbl)
if not tbl then return {} end
local hash = {}
for k, v in pairs(tbl) do
hash[v] = k
end
return hash
end
function p.arrayHash(tbl)
if not tbl then return {} end
local hash = {}
for k, v in ipairs(tbl) do
hash[v] = k
end
return hash
end
function p.appendHash(parent, tbl)
for k, v in pairs(tbl) do
parent[v] = k
end
return parent
end
function p.sortByKeyOrder(tblToSort,values)
-- sorts tblToSort to be in the same order as the elements appear in lookup
local lookup = p.hash(values)
table.sort(tblToSort, function (a,b)
return (lookup[a] or 0) < (lookup[b] or 0)
end
)
return
end
function p.uniqueArray(tbl)
local hash = {}
local ret = {}
for _, v in ipairs(tbl) do
if not hash[v] then
hash[v] = true
ret[#ret+1] = v
end
end
return ret
end
function p.sortUnique(tbl)
table.sort(tbl)
local tbl2 = {}
local i = 0
for k, v in ipairs(tbl) do
if v ~= tbl2[i] then
i = i + 1
tbl2[i] = v
end
end
return tbl2
end
function p.mergeArrays(tbl1, ...)
-- tbl1 is modified to include the elements of tbl2 appended to the end. Order is preserved.
if not tbl1 then tbl1 = {} end
local newTables = {...}
for _, tbl2 in ipairs(newTables) do
for _, v in ipairs(tbl2) do
tbl1[#tbl1+1] = v
end
end
return tbl1
end
function p.uniqueKeyMergeArrays(key, tbl1, ...)
-- tbl1 is modified to include the elements of tbl2 appended to the end. Order is preserved.
-- skips rows in later tables that have repeat values of a key.
-- useful when unioning Cargo tables with a net groupBy.
if not tbl1 then tbl1 = {} end
local newTables = {...}
local seenKeys = {}
for _, tbl2 in ipairs(newTables) do
for _, row in ipairs(tbl2) do
if not seenKeys[row[key]] then
seenKeys[row[key]] = true
tbl1[#tbl1+1] = row
end
end
end
return tbl1
end
function p.merge(tbl1, ...)
-- tbl1 is modified to include all the elements of tbl2.
if not tbl1 then tbl1 = {} end
local tables = {...}
for _, tbl2 in ipairs(tables) do
for k, v in pairs(tbl2) do
tbl1[k] = v
end
end
return tbl1
end
function p.mergeDontOverwrite(tbl1, ...)
-- tbl1 is modified to include all the elements of tbl2.
if not tbl1 then tbl1 = {} end
local tables = {...}
for _, tbl2 in ipairs(tables) do
for k, v in pairs(tbl2) do
if tbl1[k] == nil then
tbl1[k] = v
end
end
end
return tbl1
end
function p.mergeAndConcat(tbl1, sep, ...)
-- tbl1 is modified to include all the elements of tbl2.
if not tbl1 then tbl1 = {} end
local tables = {...}
for _, tbl2 in ipairs(tables) do
for k, v in pairs(tbl2) do
if not tbl1[k] then
tbl1[k] = v
else
tbl1[k] = ('%s%s%s'):format(tbl1[k], sep, v)
end
end
end
return tbl1
end
function p.mergeDicts(tbl1, ...)
-- tbl1 is modified to include the elements of tbl2 appended to the end. Order is preserved.
if not tbl1 then tbl1 = {} end
local newTables = {...}
for _, tbl2 in ipairs(newTables) do
for _, v in ipairs(tbl2) do
tbl1[#tbl1+1] = v
tbl1[v] = tbl2[v]
end
end
return tbl1
end
function p.remove(tbl, key)
-- table.remove for non-numerical key
local output = tbl[key]
tbl[key] = nil
return output
end
function p.removeValue(tbl, val)
for k, v in pairs(tbl) do
if val == v then
tbl[k] = nil
end
end
return tbl
end
function p.removeValueFromArray(tbl, val)
local len = #tbl
for i = len, 1, -1 do
if tbl[i] == val then
table.remove(tbl, i)
end
end
end
function p.removeDuplicates(tbl)
local hash = {}
local ret = {}
for _, v in ipairs(tbl) do
if not hash[v] then
hash[v] = true
ret[#ret+1] = v
end
end
return ret
end
function p.removeDuplicateKeys(tbl, key)
-- assumes tbl is an array of tables (rows) that all contain some key `key`
-- edits the original table in place to keep the first copy of each row
-- also returns the table
local hash = {}
local tbl2 = {}
for _, row in ipairs(tbl) do
if not hash[row[key]] then
hash[row[key]] = true
tbl2[#tbl2+1] = row
end
end
for i, _ in ipairs(tbl) do
tbl[i] = tbl2[i]
end
return tbl
end
function p.reverse(tbl)
-- returns a copy of tbl with the elements in opposite order (not a deep copy)
local tbl2 = {}
local len = #tbl
for i = len, 1, -1 do
tbl2[len - i + 1] = tbl[i]
end
return tbl2
end
function p.reverseInPlace(tbl)
local len = #tbl
local stop_at = len / 2
for i = 1, stop_at do
local temp = tbl[i]
tbl[i] = tbl[len - i + 1]
tbl[len - i + 1] = temp
end
end
function p.shallowClone(tbl)
-- mostly to be able to use # operator on something from mw.loadData
local tbl2 = {}
for k, v in pairs(tbl) do
tbl2[k] = v
end
return tbl2
end
function p.slice(tbl, s, e)
if s < 0 then
s = #tbl + 1 + s
end
if e < 0 then
e = #tbl + 1 + e
end
local tbl2 = {}
for k = s, e do
tbl2[#tbl2+1] = tbl[k]
end
return tbl2
end
function p.printList(tbl)
-- prints the table as a comma-separated list with and
if #tbl == 1 then
return tbl[1]
elseif #tbl == 2 then
return table.concat(tbl, ' and ')
else
last = table.remove(tbl, #tbl)
list = table.concat(tbl, ', ')
return list .. ', and ' .. (last or '')
end
end
function p.removeFalseEntries(tbl, max)
if not max then
max = 1
for _, _ in pairs(tbl) do
max = max + 1
end
end
local j = 0
for i = 1, max do
if tbl[i] then
j = j + 1
tbl[j] = tbl[i]
end
end
for i = j+1, max do
tbl[i] = nil
end
return tbl
end
function p.padFalseEntries(tbl, max, default)
default = default or ''
for i = 1, max do
if not tbl[i] then
tbl[i] = default
end
end
return tbl
end
function p.concat(tbl, sep, f, ...)
if not tbl then return end
if not sep then sep = ',' end
if not f then
local tbl2 = mw.clone(tbl)
p.removeFalseEntries(tbl2)
return table.concat(tbl2, sep)
end
local tbl2 = {}
for k, v in ipairs(tbl) do
if v then
tbl2[#tbl2+1] = f(v, ...)
end
end
return table.concat(tbl2, sep)
end
function p.concatDict(tbl, sep, f, ...)
local tbl2 = {}
for _, v in ipairs(tbl) do
tbl2[#tbl2+1] = tbl[v]
end
return p.concat(tbl2, sep, f, ...)
end
function p.concatNonempty(tbl, sep, f, ...)
if not tbl then return end
if not next(tbl) then return end
return p.concat(tbl, sep, f, ...)
end
function p.concatFromArgs(args, argname, sep, f)
-- if fields are saved in args as field1, field2, field3, etc
local i = 1
local tbl = {}
if args[argname] then
tbl[1] = args[argname]
i = 2
end
while args[argname .. i] do
tbl[i] = args[argname .. i]
i = i + 1
end
return next(tbl) and p.concat(tbl, sep, f)
end
function p.crop(tbl, max)
for k, _ in ipairs(tbl) do
if k > max then
tbl[k] = nil
end
end
end
function p.alphabetize(tbl)
local hash = {}
local nocase = {}
for i, v in ipairs(tbl) do
nocase[i] = lang:caseFold(v)
hash[nocase[i]] = v
end
table.sort(nocase)
for i, v in ipairs(nocase) do
tbl[i] = hash[v]
end
return tbl
end
function p.guaranteeTable(c, f, ...)
if not c then return nil end
if type(c) == 'table' then
return f and f(c, ...) or c
end
return { f and f(c, ...) or c }
end
function p.guaranteeIndex(tbl, i)
if type(i) == 'number' then return i end
return p.keyOf(tbl, i)
end
function p.concatIfTable(tbl, sep)
if not tbl then return nil end
return p.concat(p.guaranteeTable(tbl), sep)
end
function p.nextNonFalse(tbl)
for _, v in pairs(tbl) do
if v then return v end
end
end
function p.interlace(tbl)
local ret = {}
local _, keyList = next(tbl)
for key, _ in pairs(keyList) do
ret[key] = {}
end
for k, v in pairs(tbl) do
for key, _ in pairs(keyList) do
ret[key][k] = v[key]
end
end
return ret
end
function p.initTable(tbl, key, val)
if tbl[key] then return end
tbl[key] = val or {}
return tbl[key]
end
function p.initDict(tbl, key, val)
if tbl[key] then return end
tbl[key] = val or {}
tbl[#tbl+1] = key
end
function p.push(tbl, val)
-- useful just to make code look cleaner when tbl is something like parent[row.key]
tbl[#tbl+1] = val
end
function p.pushDict(tbl, key, val)
tbl[#tbl+1] = key
tbl[key] = val
end
function p.arrayToDict(tbl, key)
for i, v in ipairs(tbl) do
tbl[v[key]] = v
tbl[i] = v
end
end
function p.extractValueToList(tbl, key)
local ret = {}
for _, v in ipairs(tbl) do
ret[#ret+1] = v[key]
end
return ret
end
function p.extractValueFromDictToList(tbl, key)
local ret = {}
for _, v in ipairs(tbl) do
ret[#ret+1] = tbl[v][key]
end
return ret
end
function p.arrayFromField(tbl, key)
local ret = {}
for k, row in ipairs(tbl) do
ret[#ret+1] = row[key]
end
return ret
end
function p.range(a, b)
local ret = {}
for i = a, b do
ret[#ret+1] = i
end
return ret
end
return p
|
You will define the preloads you want at subpages of Module:CharInserts
, named for the namespace they belong to. Here is an example module that you could use at Module:CharInserts/Template
:
Module:CharInserts/Template
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
|
-- <nowiki>
return {
{
pattern = '',
label = 'insert includeonly',
notpattern = '/doc$',
insert = [[<includeonly>+</includeonly><noinclude>{{documentation}}</noinclude>]],
},
{
pattern = '/CargoAttach$',
label = 'Insert Attach Prefill',
insert = [[<includeonly></includeonly><noinclude>{{documentation
|cargodec={{Attach|{{subst:BASEPAGENAME}}}}
\[\[Category:Fake Cargo Attach Templates\]\]+
}}</noinclude>
]]
},
{
pattern = '',
notpattern = '/CargoAttach',
label = 'Insert DRUID infobox',
insert = [[<includeonly>{{#invoke:Infobox|main
|kind=
|sep=,
|image={{#if:{{{image|}}}|[\[File:{{{image}}}{{!}}300px\]\]}}
|sections=
+
}}</includeonly><noinclude>{{documentation}}</noinclude>]]
}
}
-- </nowiki>
|
Interface pages
Finally, we will need to show the charinserts when editing pages.
For each namespace number N
that you want to display CharInserts at, add the following code at MediaWiki:editnotice-N
:
{{#invoke:CharInserts|main}}
For example, if you want CharInserts in the main namespace (0), the user namespace (2), and the template namespace (10), you would create these pages:
Mediawiki:editnotice-0
Mediawiki:editnotice-2
Mediawiki:editnotice-10
What interface pages to make?
Interlude: Finding system messages
If you add the argument uselang=qqx
to the URL of any page (if there’s already one or more specified params, put &uselang=qqx
; if not, then ?uselang=qqx
) will show you the names of system messages instead of their contents.
So, say I edit my user page on Leaguepedia. Normally the url is https://lol.gamepedia.com/index.php?title=User:RheingoldRiver&action=edit
, but I want to see what system messages are present, so I’ll instead go to https://lol.gamepedia.com/index.php?title=User:RheingoldRiver&action=edit&uselang=qqx
. (Note that the ?
comes earlier in the URL, before the first parameter, which is title=User:RheingoldRiver
.)
Back to deploying CharInserts
So on my user page, I’m shown the following four system message names:
(editnotice-2)
(editnotice-2-RheingoldRiver)
(longpage-hint: (size-kilobytes), 1115)
(editpage-head-copy-warn)
The last one is included on every page on the entire wiki, but I’d rather avoid using the copyright warning to display editor tools. Instead I’m going to use the first message, which is editnotice-2
. If I get a list of all of my namespaces (doable either by going manually through Special:AllPages
or using the API), I can get a list of system messages I will have to display:
MediaWiki:Editnotice-0
(main)
MediaWiki:Editnotice-1
(talk)
MediaWiki:Editnotice-2
(user)
MediaWiki:Editnotice-3
(user talk)
- etc.
I’m going to place the same text on all of them, except for the first:
{{int:Editnotice-0}}
This internationalizes (translates) the edit notice for the main namespace and displays it on each other namespace’s edit notice.
At MediaWiki:Editnotice-0
I have the following:
Thanks for editing the wiki! Want to join our Discord server? {{DiscordURL}}
{{#invoke:CharInserts|main}}
The first line is unrelated to CharInsert; the second simply invokes my CharInserts module.
As it happens, I actually want my CharInserts to behave differently depending on namespace - I have some preloads that are template-only, some that are main-only, etc. However, I chose to do all of this handling inside of Lua, so in the system messages I’m merely invoking the same module on all pages.
Lua config file setup
As I mentioned, I have different behaviors depending on namespace. I also have different behaviors depending on pattern matches against the title of the current page. In particular, the following parameters are provided for each insert:
pattern
, a required pattern to appear somewhere in the title; if this is the empty string, then every title matches it by default;
notpattern
, a pattern or list of patterns that must NOT appear in the title if the insert is to appear; this takes precedence over pattern
;
label
, the human-readable text to display for an editor to click; and
insert
, the actual text (human-readable and unescaped) that the CharInsert will add to the page.
Because often notpattern
is a list of “pages with patterns that are handled specially,” at the start of most config files I define a constant called NOT_PATTERNS
that will be used as the notpattern
for many inserts. (This is also why config files as code are better than standalone non-code text files.)
Here is an excerpt from my Main
namespace CharInserts. Note that indentation style is not preserved mid-insert to avoid sending careless whitespace to the charinsert. At the same time, I deliberately arranged insert
to be the final item in each group, so that I didn’t need to change indentation after it.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
local NOT_PATTERNS = {
"/Team Rosters",
"/Casting History",
}
return {
{
pattern = '/Casting History',
label = "Insert Casting History",
insert = [[{{PlayerTabsHeader}}
{{CastingHistory}}]]
},
{
pattern = "",
notpattern = NOT_PATTERNS,
label = "Insert skin page",
insert = [[{{Infobox Skin
|champion=
|name=
|rp=
|date=
|legacy=
|artists=
|artistlinks=
}}
{{ChampionSkinIntro}}
{{ChampionSkinImageSections}}
]]
},
{
pattern = ' Teams$',
label = "Insert TeamCountryPortal",
insert = "{{TeamCountryPortal|+}}"
},
{
pattern = ' Teams$',
label = "Insert TeamRegionPortal",
insert = "{{TeamRegionPortal|+}}"
},
{
pattern = '/Scoreboards',
label = "Insert Scoreboard/Tab",
insert = [[{{Scoreboard/Tab|+|continue=}}]]
},
}
|
Pagename
I want to point one final thing out about the peculiarities of escaping for CharInsert: Normally, if I want the title of a page inserted into that page, I can simply used {{subst:PAGENAME}}
or {{subst:BASEPAGENAME}}
or what have you. However, subst
does NOT work inside of <includeonly>
tags.
Therefore, for one of my inserts for the Template
namespace, when I want to invoke a Lua module with the same title as the template, I write the following:
<includeonly>{{#invoke:$PAGENAME$|main}}</includeonly><noinclude>{{documentation}}</noinclude>
the string $PAGENAME$
follows other conventions for formatting parameters into longer strings that I use elsewhere on the wiki, so part of my escaping/formatting is the following:
1
2
3
4
5
6
|
function h.makeReplacements(str)
local replacements = {
PAGENAME = mw.title.getCurrentTitle().text,
}
return util_sentence.makeReplacements(str, replacements)
end
|
And the code in Module:SentenceUtil
for makeReplacements
is as follows:
1
2
3
4
5
6
7
8
9
10
11
12
13
|
function p.makeReplacements(sentence, replacements)
if not sentence then return nil end
if not replacements then replacements = {} end
local old = sentence
for k, v in pairs(replacements) do
sentence = sentence:gsub('%$' .. k .. '%$', v or 'unknown')
end
if sentence == old then
return sentence
end
return p.makeReplacements(sentence, replacements)
end
|
(Note the recursive capacity of replacements
- while it’s not needed here, in other situations, it’s required.)
In the future, if I find other necessary pre-printing substitutions to make, I can simply append additional keys to the table replacements
in the first snippet above.
Conclusion
CharInserts is a really nice compromise between VE and plain wikitext, the other main contender (imo) being PageForms. With a bit of Lua code you can easily customize CharInserts to offer as many different preloads as you like.
Since publication of this post, CharInserts has finally added natively the ability to make display text & inserted text different; this code doesn’t take advantage of that functionality. If you wanted to, you could probably adjust the Lua to output the HTML they expect and not import any special CSS and JS.